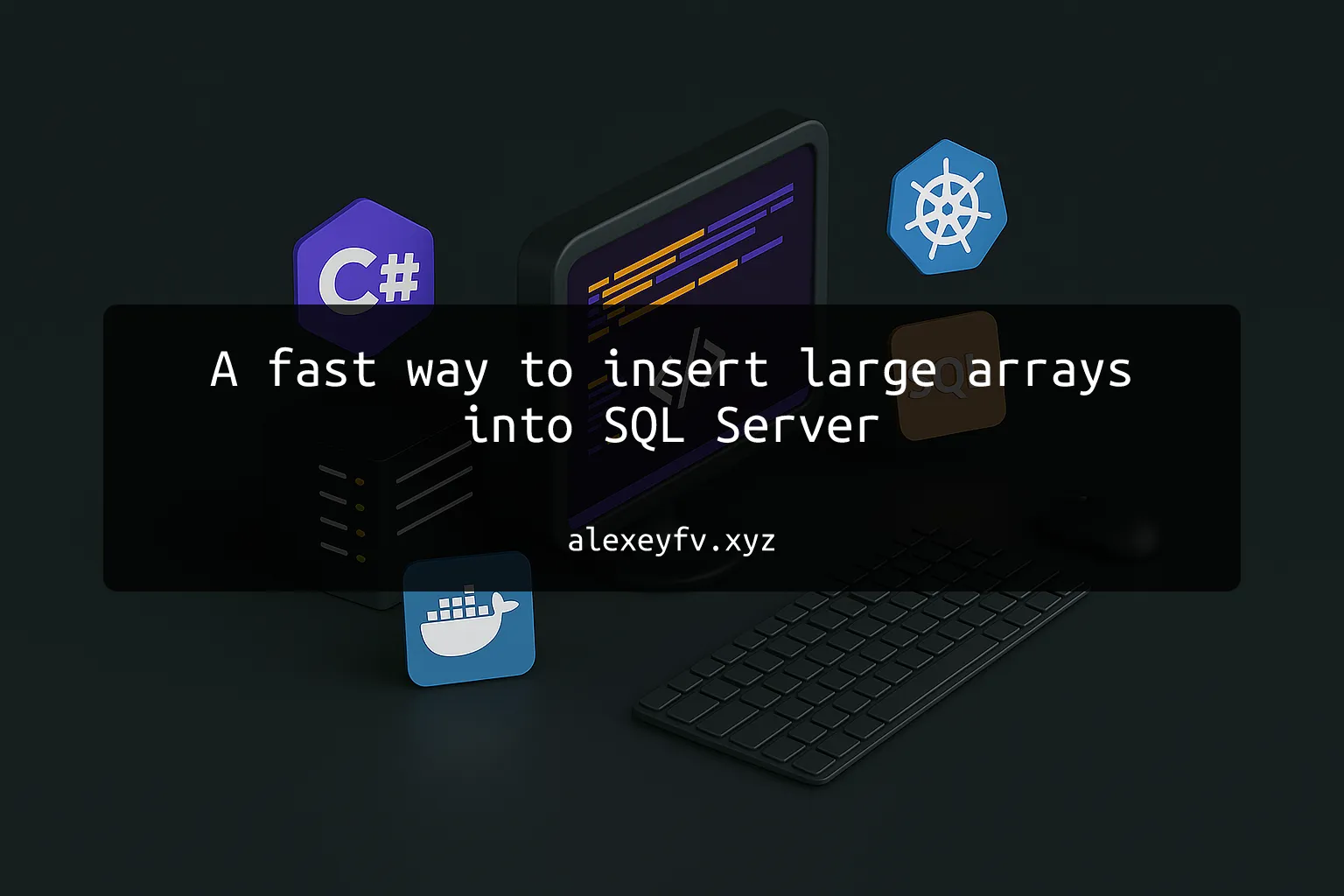
A fast way to insert large arrays into SQL Server
How to quickly and efficiently load large data into SQL Server: comparing regular INSERT, SqlBulkCopy with DataTable, and IDataReader.
-->
How to quickly and efficiently load large data into SQL Server: comparing regular INSERT, SqlBulkCopy with DataTable, and IDataReader.
Analysis of CA1830 code quality rule and its impact on application performance
Analysis of CA1826 code quality rule and its impact on application performance
How to use xxHash in C# for fast, stable hash computation without allocations
How logging in C# affects application performance. Comparing string interpolation, templates, and LoggerMessageAttribute.
C# duck typing patterns with foreach loops, async-await, and collection initialization syntax
How to reduce boilerplate code in EventFlow library using C# Source Generators
A deep dive into performance and internal implementation details of Object Pool pattern in C#
A deep dive into performance and internal implementation details of FrozenDictionary in .NET 8
A short experiment on how array initialization methods impact performance
Common mistakes in writing benchmarks in C# and how to fix them
Analyzing the hash collision counting algorithm in FrozenDictionary and its optimization using bitwise operations
A performance and applicability comparison of seven different ways to create arrays in C#
An analysis of the optimized modulo calculation algorithm used in FrozenDictionary, compared to the classic approach
Optimizing Dictionary performance in C# using structs and CollectionsMarshal
Integration testing in C# using Testcontainers
Detailed exploration of the performance differences between C# structures and classes
Analysis of the limitations of the Options pattern in C#
A performance comparison between ReadOnlySpan<T> and string operations in C#
A performance comparison of different substring extraction methods in C#
Investigating performance issues when passing a method as a parameter in C#
A guide on how to call the Main method in C# programs that use top-level statements
A guide to improving your code with Domain-Driven Design and Rich Domain Model
A performance comparison of different EF mapping strategies in SQL Server
Step-by-step guide to building and configuring a blog using Jekyll and GitHub Pages
Data types, functions, and currying in F#
An example of how to implement the Fibonacci sequence in F# and C#
Limitations and issues with enums in .NET, and how to solve them using the enum class pattern